第二篇主要是 react react-router react-redux
React
|
|
|
|
运行可见 Hello React!
React-router
|
|
Home 组件:
|
|
React-redux
|
|
src 文件夹下新建 store 文件夹,并创建 index.js 以及 reducer.js
|
|
修改入口文件 index.js 以及 app.js(分离出了一个app.js,看上去河蟹一些)
|
|
通过 Provider 的 store 属性把状态暴露给各个组件方便集中管理使用
接下来实现 Counter 组件
src 目录下的 container 文件夹下 新建 Counter 文件夹,并在文件下下建立如下文件:
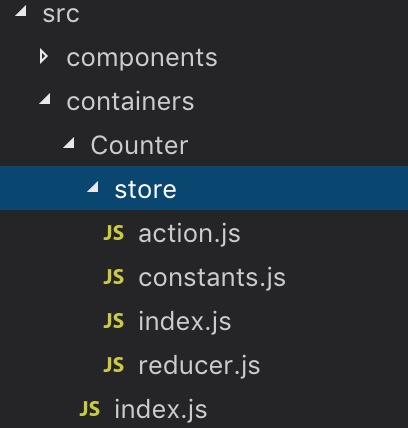
|
|
然后编写代码:
定义行为变量 constants ,包含三态:自增、自减以及重置
|
|
定义 reducer ,即我们触发 action 会改变 store 里的状态
|
|
定义我们的 action
|
|
集体导出统一管理:(供根目录的combineReducers消费)
|
|
接下来修改 Counter 组件
|
|
Redux-thunk
redux-thunk 使得 action 可以返回一个函数组为返回值
|
|
同理,在 container 文件夹下创建如下目录结构:
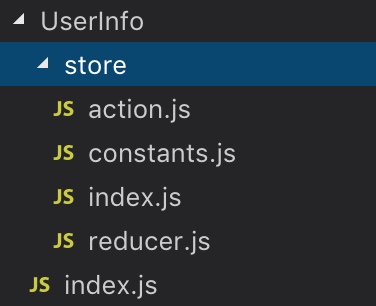
接下来是类似的代码:
|
|
最后偷个图:
自己连抄带查,搞定了一个脚手架,记录一下。
学习就是不断做一件事1000遍以上的过程,加油。